Java Random Nextint Always 0
2
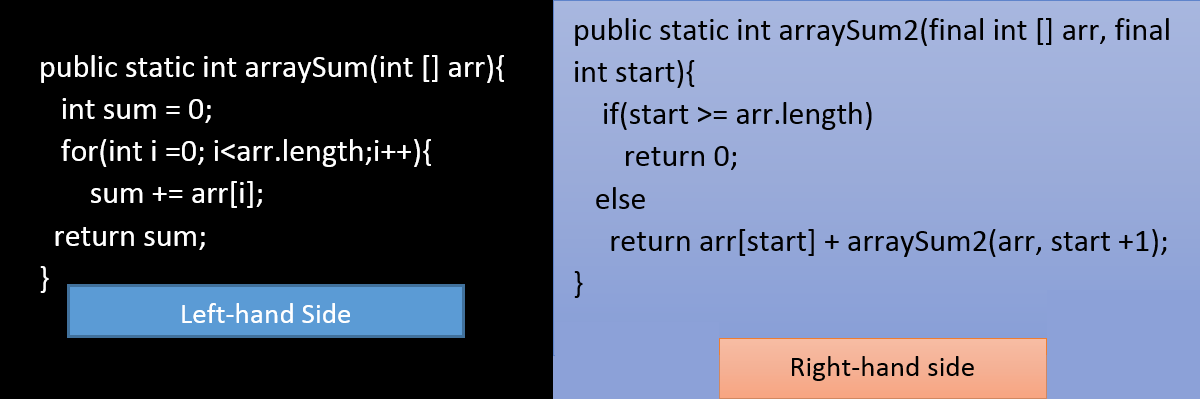
Anatomy Of Sequential Data Processing With Java Streams Dzone Java
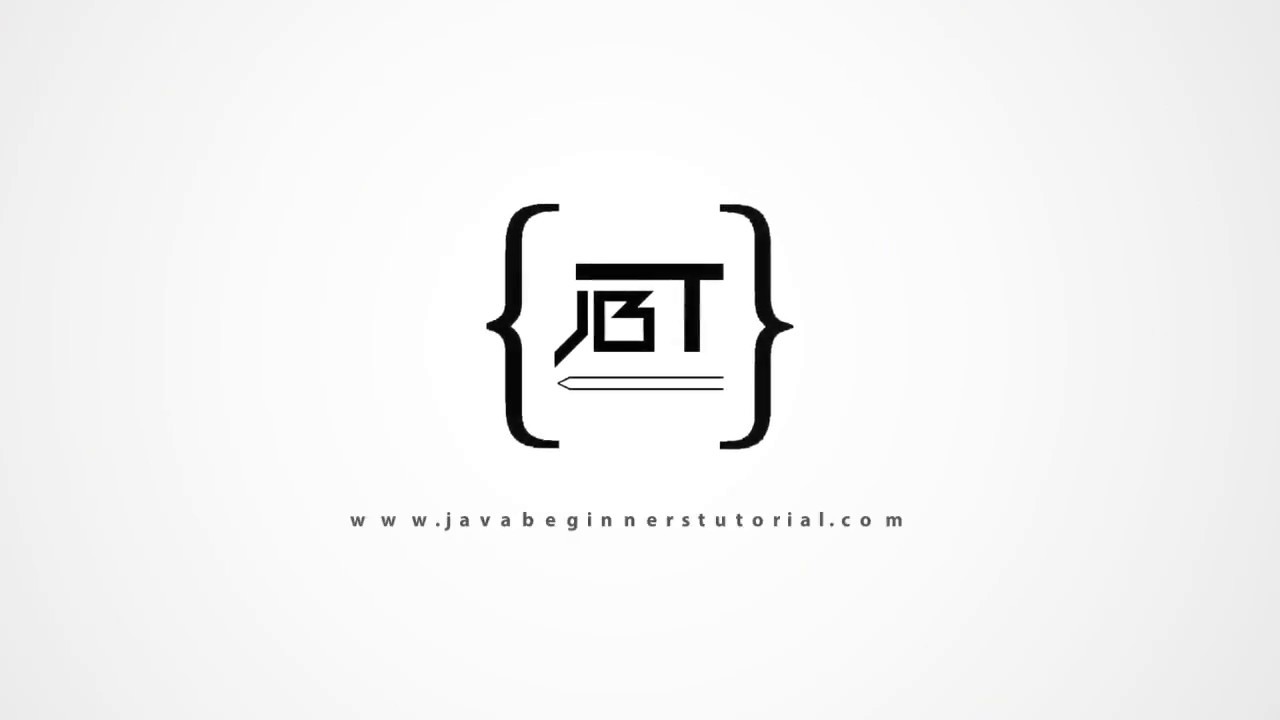
Generate Random Number In Java Java Beginners Tutorial
2
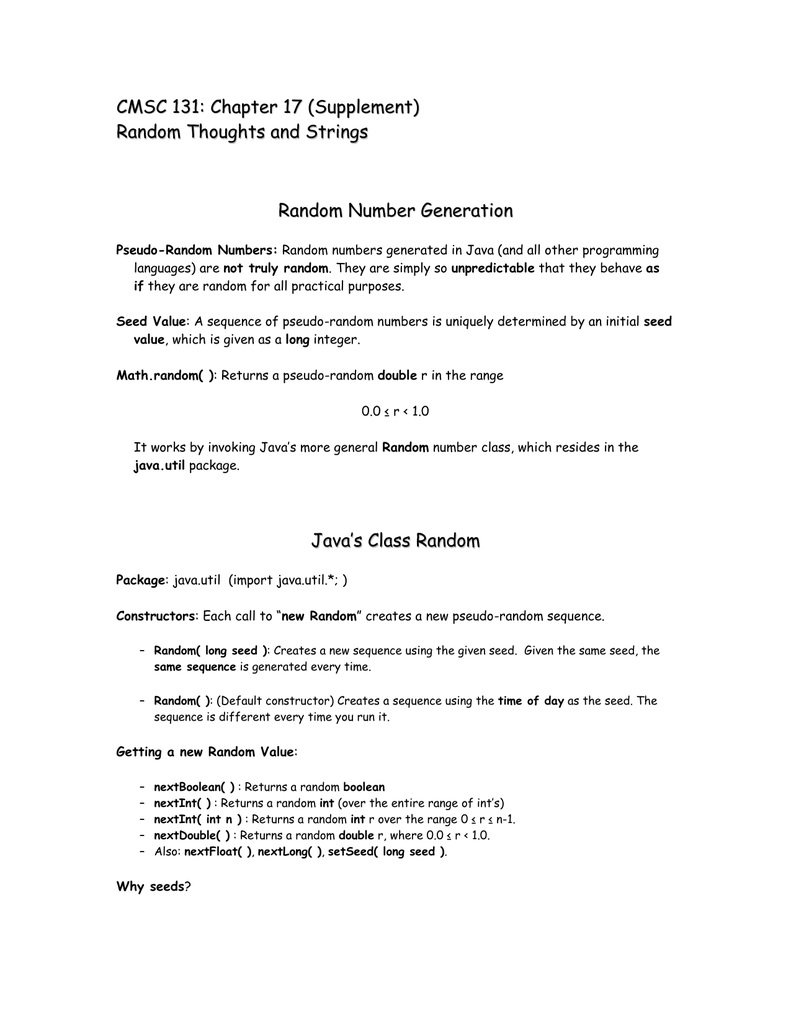
C M S

Java Random Journaldev
For example, methods nextInt() and nextLong() will return a number that is within the range of values (negative and positive) of the int and long data.

Java random nextint always 0. Let’s have a quick look at the example:. NextLong () Returns the next pseudorandom, uniformly distributed long value from this random number generator's sequence. If you need a cryptographically secure random generator – use java.
The java.util.Random class generates random integers, doubles, longs and so on, in various ranges. During debugging, it is often useful to set the internal seed for the random generator explicitly so that it always returns the same sequence. Java 8 also adds the nextGaussian () method to generate the next normally-distributed value with a 0.0 mean and 1.0 standard deviation from the generator's sequence.
The new license permits certain uses, such as personal use and development use, at no cost -- but other uses authorized under prior Oracle Java licenses. That should give a distribution between 0.0 and 1.0 in steps of approx 0. Using java.util.Random to generate random numbers.
For any precision write your own Random class or try this. This class provides additional methods for generating random streams, that employ the above techniques when used in stream.parallel() mode. Math Random Java OR java.lang.Math.random() returns double type number.
The integer returned by nextInt could be any valid integer (positive or negative) whereas the number returned by nextFloat is a floating point value between 0 and 1 (up to but not including the 1). This subclass of java.util.Random adds extra methods useful for testing purposes. In order to generate random array of integers in Java, we use the nextInt() method of the java.util.Random class.
Thus, when we convert the multiplication to an int value, as I have done in the example, it will end up being 1 less than the size specified.For example, if the list size is 5 and the random number is 0.9999, the multiplication will. RandomObject (nextInt () * 1.0 / .0. Random rand = new Random();.
* For simple random doubles between 0.0 and 1.0, you may consider using 68:. I < 10 ;. Returns the next pseudorandom, uniformly distributed int value from this random number generator’s sequence Syntax:.
Java.util.Random.nextInt (int n) :. The following examples show how to use java.security.SecureRandom#nextInt() .These examples are extracted from open source projects. Following is the declaration for java.util.Random.nextDouble() method.
/** * Create a random number that is greater than or equal to 0, * and less than 100. Min + random.nextInt (max – min + 1). For (int i = 0 ;.
Int result = new intSIZE;. It doesn’t take any parameter and simply returns a number which is greater than or equal 0.0 and less than 1.0. This method returns a pseudorandom positive double value between 0.0 and 1.0, where 0.0 is inclusive and 1.0 is exclusive.
In this post, we will see how to generate random numbers between 0 to n, both inclusive, in Java using Random class, Math.random(), ThreadLocalRandom class, SecureRandom class, and Apache Commons. This will provide a random number based on the argument specified as the upper limit, whereas it takes lower limit is 0.Thus, we get 10 random numbers displayed. The nextDouble() method is used to get the next pseudorandom, uniformly distributed double value between 0.0 and 1.0 from this random number generator's sequence.
Generate random numbers between 0 to N. It means Math.random() always return a number greater than or equal to 0.0 and less than 1.0. A new pseudorandom-number generator, when the first time random() method called.
Each invocation of this method returns a random number. An object of Random class is initialized as objGenerator. The nextInt (int n) is used to get a random number between 0 (inclusive) and the number passed in this argument (n), exclusive.
Internally it uses java.util.Random class. In comparison to other methods, Math.random () only returns Double values. Java - Random nextInt() Method The java.util.Random.nextInt() method is used to return a pseudorandom, uniformly distributed int value between 0 (inclusive) and the specified value (exclusive), drawn from this random number generator's sequence.
Normally, you might generate a new random number by calling nextInt(), nextDouble(), or one of the other generation methods provided by Random.Normally, this intentionally makes your code behave in a random way, which may make it harder to test. The Randomly generated integer is :. // randomNumber has a random value between 0 and 9 Method name Description nextInt() returns a random integer nextInt(max) returns a random integer in the range 0, max) in other words, 0 to max-1 inclusive.
* @ author Eric Blake (ebb9@email.byu.edu) 74:. Class Randomis found in the java.utilpackage. For example, a fork/join-style computation using random numbers might include a construction of the form new Subtask(aSplittableRandom.split()).fork().
A random number generator isolated to the current thread. Scanner class and its function nextInt() is used to obtain the input, and println() function is used to print on the screen.;. Random doesn't mean "evenly scattered", it means "in a not defined-in-advance pattern".
Important Oracle Java License Update The Oracle Java License has changed for releases starting April 16, 19. A 50/50 chance isn't random, so you'll have to decide which you want. * java.util.Random codeimport java.util.Random;.
The next pseudorandom. Again a small tweak is needed. In Java, we can generate random numbers by using the java.util.Random class.
Flipping an evenly-weighted coin 100 times and getting 99 tails doesn't mean that the flip wasn't random. * @ see Math#random() 72:. To do so, you need to invoke the setSeed method.
Random.nextInt(bound) Here random is object of the java.util.Random class and bound is integer upto which you want to generate random integer. 0. 3) java.util.concurrent.ThreadLocalRandom class This class is introduced in java 1.7 to generate random numbers of type integers, doubles, booleans etc. The following code generates 10 random numbers and prints them.
Once we import the Random class, we can create an object from it which gives us the ability to use random numbers. When applicable, use of ThreadLocalRandom rather than shared Random objects in concurrent programs will typically encounter much less overhead and contention. Random.nextInt() We can use nextInt() provided by Random class that returns a pseudorandomly generated int value in the specified range.
Random’s nextInt method will generate integer from 0 (inclusive) to bound (exclusive) If bound is negative then it will throw IllegalArgumentException. After it used thereafter for. The next pseudorandom, Gaussian ("normally") distributed double value with mean 0.0 and standard deviation 1.0 from this random number generator's sequence java.util.Random.nextInt():.
This Java program asks the user to provide maximum range, and generates a number within the range. Like the global Random generator used by the Math class, a ThreadLocalRandom is initialized with an internally generated seed that may not otherwise be modified. (Randomly, your program could return 1 99 times and it would still be random.
Declaration − The java.util.Random.nextInt() method is declared as follows − public int nextInt(). There is two different types of Java nextInt() method which can be differentiated depending on its parameter. Public int nextInt() Returns:.
In the below example, the java.util.Random.nextInt() method is used to get pseudorandom number, uniformly distributed int value. The method call returns the next pseudorandom, uniformly distributed double. They are designed to be split, not shared, across threads.
To generate random numbers, you construct an object of q the class Random, and then use the method nextInt(n) which returns a number between 0 (inclusive) and n (exclusive). * @ see java.security.SecureRandom 71:. Returns the next pseudorandom, uniformly distributed int value from this random number generator's sequence.
Alternatively, the java.util.Random class provides methods for generating random booleans, bytes, floats, ints, longs and 'Gaussians' (doubles from a normal distribution with mean 0.0 and standard deviation 1.0). That’s why I’ll show you an. It improves performance and have less contention than Math.random() method.
Int randomNumber = rand.nextInt(10);. View Cost.java from CS 115 at Illinois Institute Of Technology. Following is the declaration for java.util.Random.nextInt() method.
Here is my code:import java.util.*;public class RandGen { Random rd;. You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example. When applicable, use of ThreadLocalRandom rather than shared Random objects in concurrent programs will typically encounter much less overhead and contention.
Where Returned values are chosen pseudorandomly with uniform distribution from that range. Let’s take a look at code examples. * @status updated to 1.4 75:.
Java - Random(seed).nextInt always generate 0 for the first number generated - Stack Overflow. Public double nextGaussian() Returns:. Static final int SIZE = 1;.
For getRandomNumberInRange (5, 10), this will generates a random integer between 5 (inclusive) and 10 (inclusive). * @ author Jochen Hoenicke 73:. Public class Random implements Serializable 77.
Public int nextInt(int n) Parameters. The general contract of nextInt is that one int value in the specified range is pseudorandomly generated and returned. Returns a pseudorandom, uniformly distributed int value between 0 (inclusive) and the specified value (exclusive), drawn from this random number generator's sequence.
Below program explains how to use this class to generate random numbers:. Random class has a lot of methods, but nextInt() is the most popular. By default, the RandomGenerator object is initialized to begin at an unpredictable point in a pseudorandom sequence.
A java.util.concurrent.ThreadLocalRandom is a utility class introduced from jdk 1.7 onwards and is useful when multiple threads or ForkJoinTasks are required to generate random numbers. We can generate random values for long and double by invoking nextLong () and nextDouble () methods in a similar way as shown in the examples above. So when you first call this method, it creates an instance of Random class and caches.
Most often the goal of a program is to generate a random integer in some particular range, say 30 to 99 (inclusive). The nextInt(int n) method is used to get a pseudorandom, uniformly distributed int value between 0 (inclusive) and the specified value (exclusive), drawn from this random number generator's sequence. The random method generates a random number that is greater than or equal to 0 and always less than 1 (i.e.
The nextInt() method of Java Scanner class is used to scan the next token of the input as an int. That is about the only way to do it I can find which doesn't have the problem of not exactly reaching 1.0. The Random class has a method as nextInt.
Returns a pseudorandom, uniformly distributed int value between 0 (inclusive) and the specified value (exclusive), drawn from this random number generator's sequence. This returns the next random integer value from this random number generator sequence. Random generates a random double number and uses Random class internally to do that.
In Java there are a few ways to do this and the two popular ways would be:. A random number generator isolated to the current thread. All n possible int values are produced with (approximately) equal probability.
Public class generateRandom{ public. Most common way of generating a random double number in Java is to use Math.random(). Default minimum number limit for Random class in "0", all you need to set is upper limit.
The nextInt () of Random class has one more variant nextInt (int bound), where we can specify the upper limit, this method returns a pseudorandom between 0 (inclusive) and specified limit (exclusive). The Random class nextInt method really does all the work in this example code. The Random class of Java library (java.util.Random) implements a random number generator.
Hello Diego, Thanks for your comment. This example shows how to generate a random number in Java that is greater than or equal to 0, and less than 100:. The new Oracle Technology Network License Agreement for Oracle Java SE is substantially different from prior Oracle Java licenses.
Random class and its function is used to generates a random number. The most basic way of generating Random Numbers in Java is to use the Math.random () method. Public double nextDouble() Parameters.
A value of this number is greater than or equal to 0.0 and less than 1.0. } This is about as simple as it gets for generating random numbers. Like the global Random generator used by the Math class, a ThreadLocalRandom is initialized with an internally generated seed that may not otherwise be modified.
For example, the following code is equivalent to that above:. The nextFloat () method is used to get the next pseudorandom, uniformly distributed float value between 0.0 and 1.0 from this random number generator's sequence.
1
Q Tbn 3aand9gcrr6ob6tb9jwykwtqbpxbtcqt2xovhexfp4yk7747wkjqifhpfu Usqp Cau
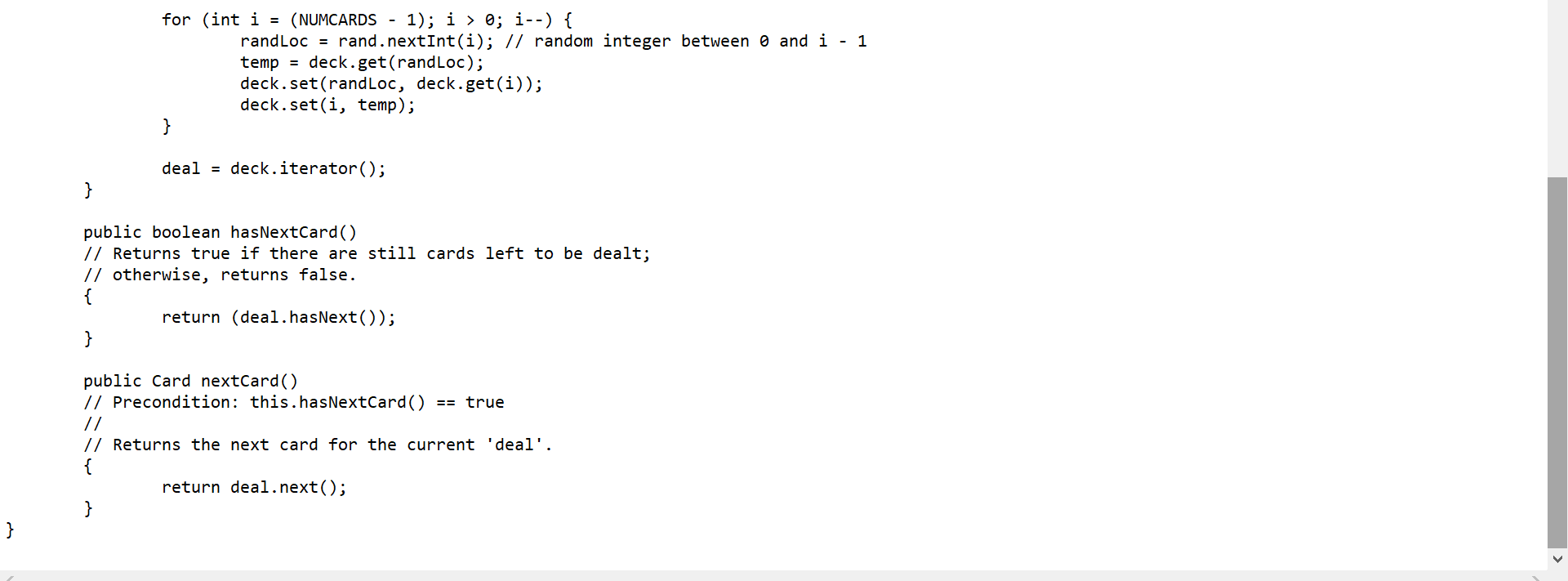
19 Add The Following Methods To The Carddeck Java Chegg Com

Build Your First Android App In Java
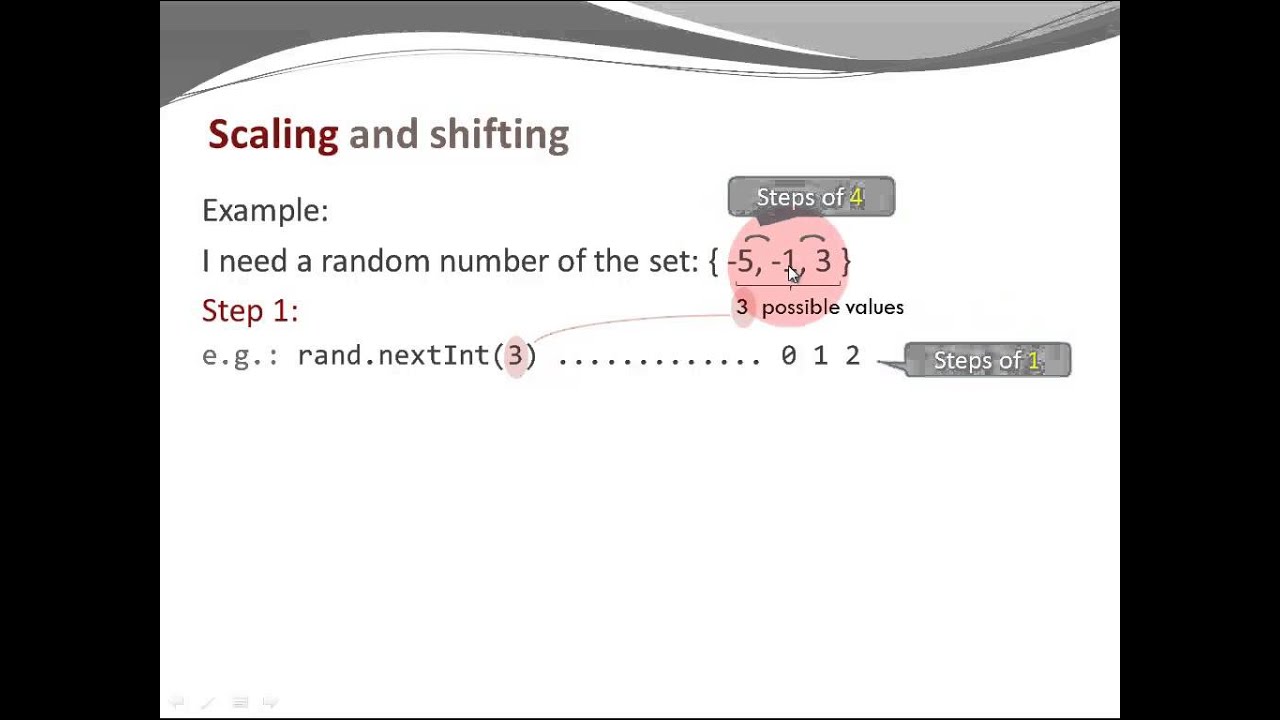
Java Basics Random Number Generation Part 2 Youtube
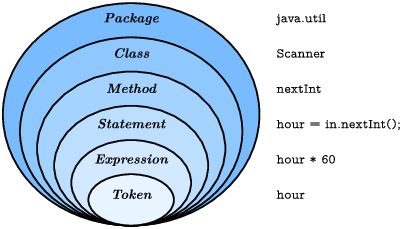
Input And Output Think Java Trinket

Building Java Programs Powerpoint Presentation Free Online Download Ppt X1qp9n

How To Create A Random Number In Java Code Example

Write A Java Program To Make A Grid Of 10x10 Matrices With 0 1 Generated Homeworklib

6 Different Ways Java Random Number Generator Generate Random Numbers Within Range
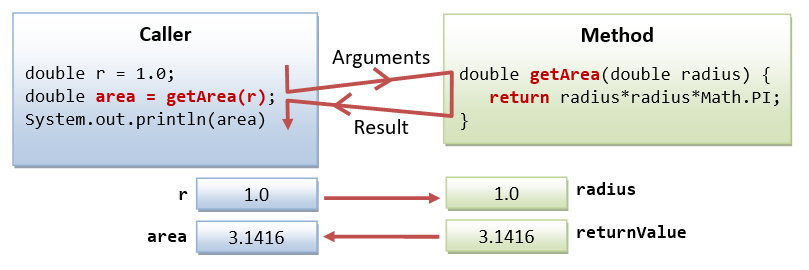
Java Basics Java Programming Tutorial

Program Is Freezed While Using Random Nextint To Much Stack Overflow

Generating Not So Random Numbers With Java S Random Class
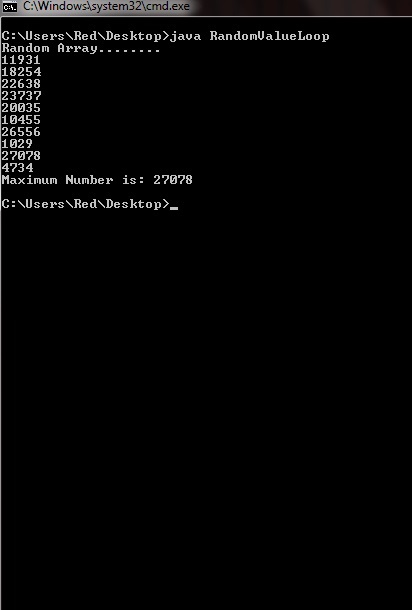
Generate Random Number Java Program Code Java Hungry
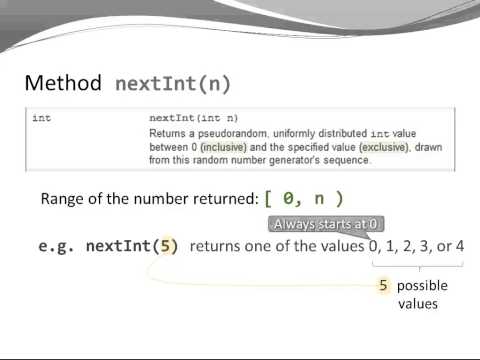
Java Basics Random Number Generation Part 1 Youtube
Linear Regression From Scratch In Java By Joker1067 Analytics Vidhya Medium

Generating Not So Random Numbers With Java S Random Class
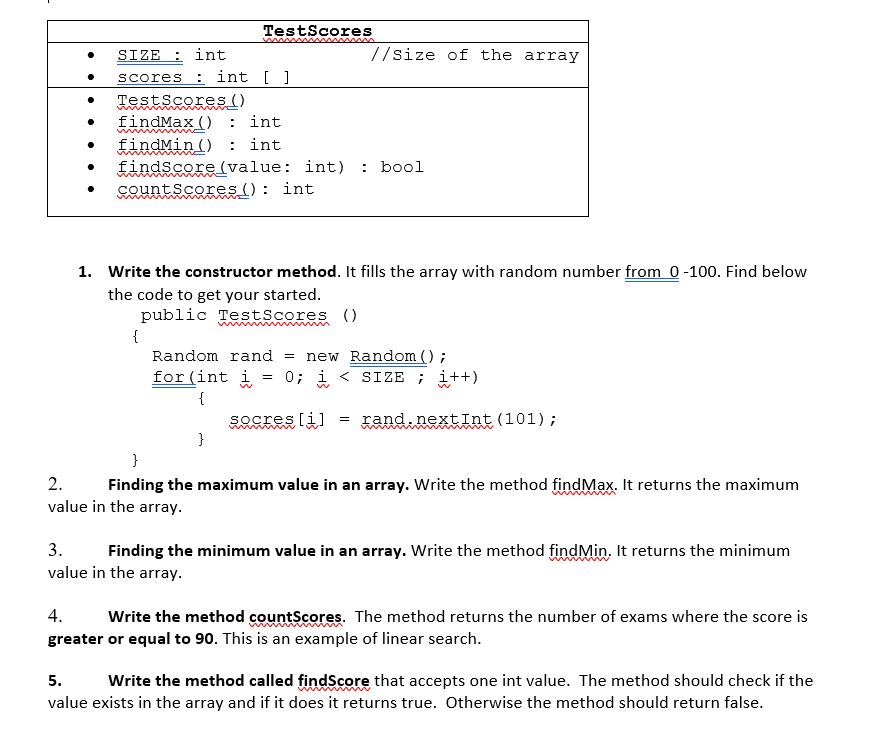
Solved Testscores Size Int Size Of The Array Scores Chegg Com
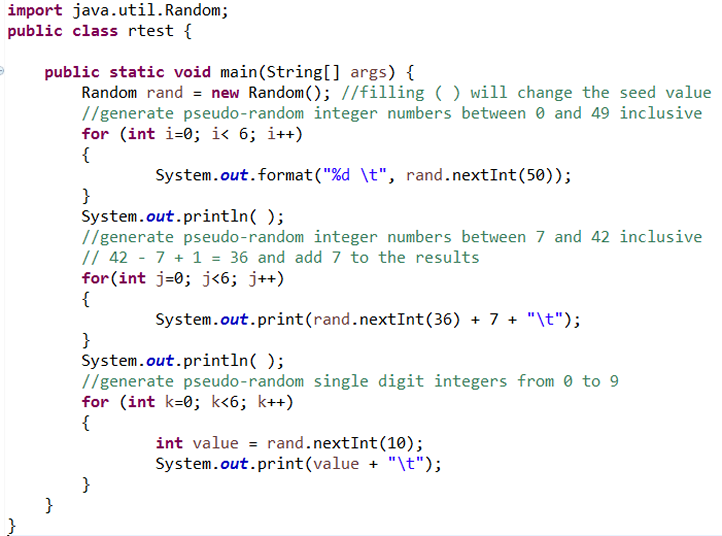
Java Random Generation Javabitsnotebook Com

Intro To Java Midterm 2 Flashcards Quizlet

Beginning Programming With Java For Dummies 4th Edition Pages 1 250 Text Version Fliphtml5

最高 Java Random Nextint

Q Tbn 3aand9gctuyax8p5o7icrxswzafihmrkudvfehnx2nsw Usqp Cau
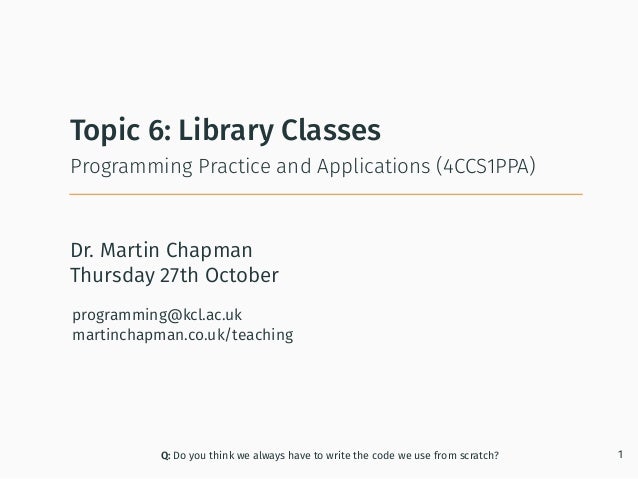
Programming In Java Library Classes
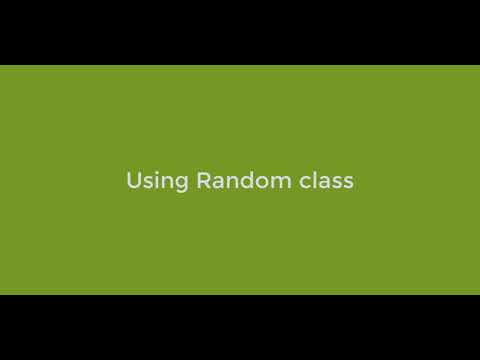
Generate A Random Number In Java In 3 Ways

Weak Random Thesecurityvault
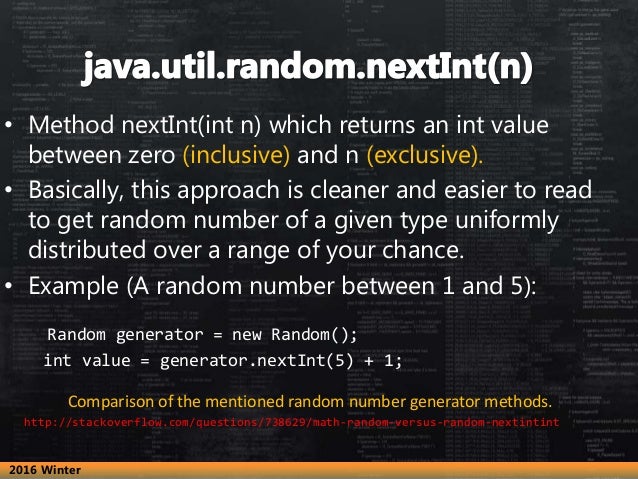
Java Tutorial Lab 2
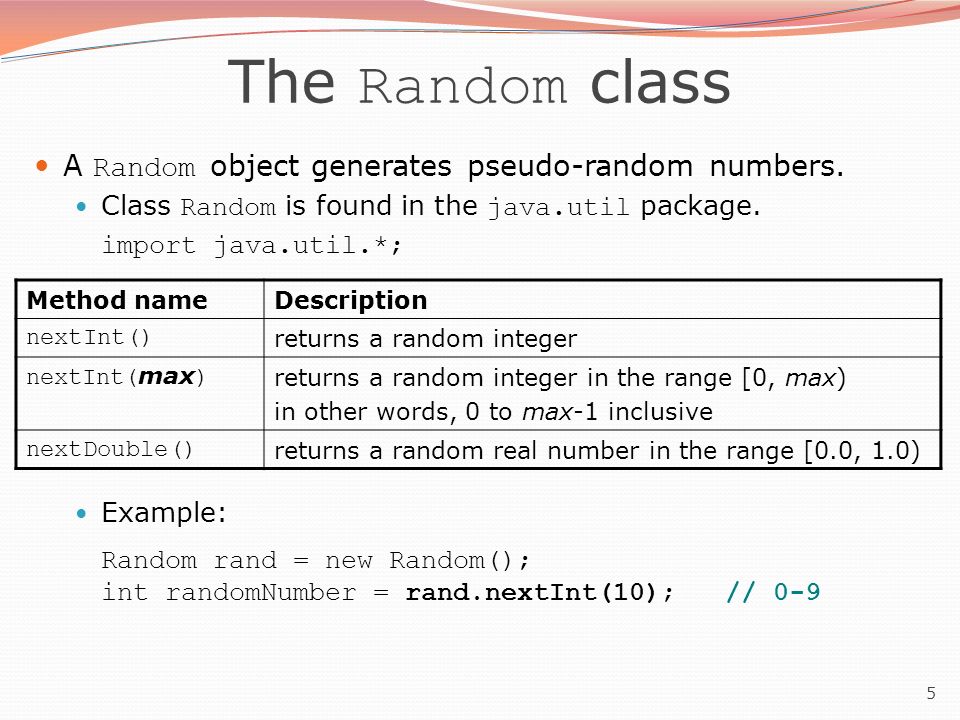
1 Building Java Programs Chapter 5 Lecture 5 2 Random Numbers Reading 5 1 Ppt Download
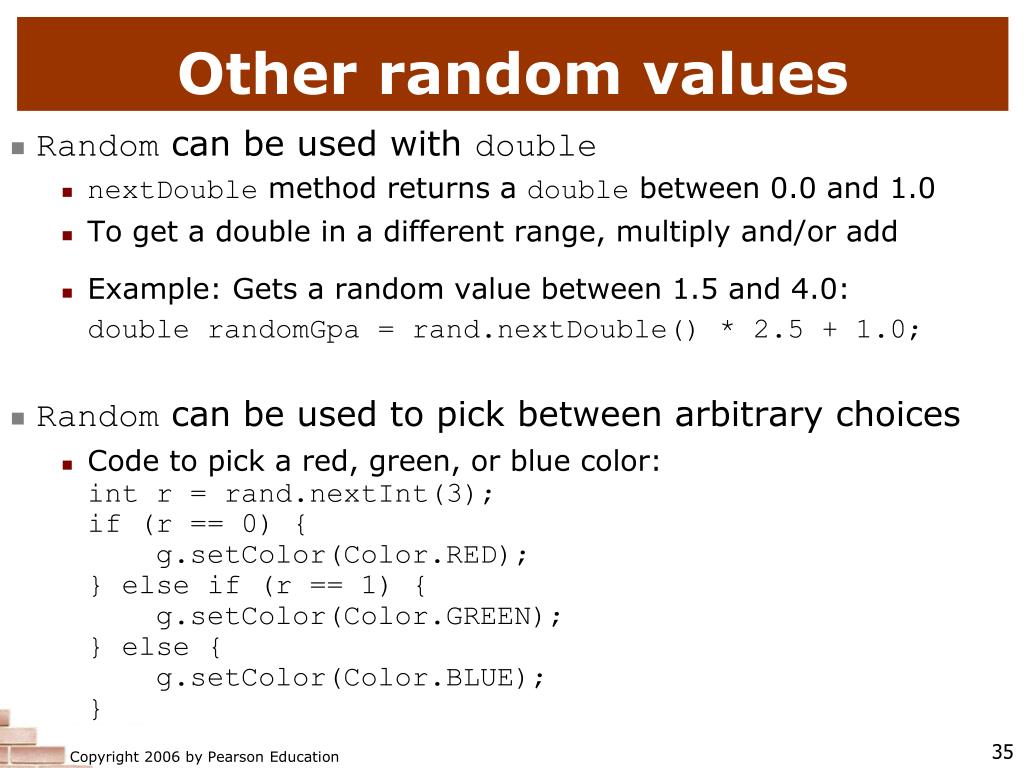
Ppt Building Java Programs Powerpoint Presentation Free Download Id
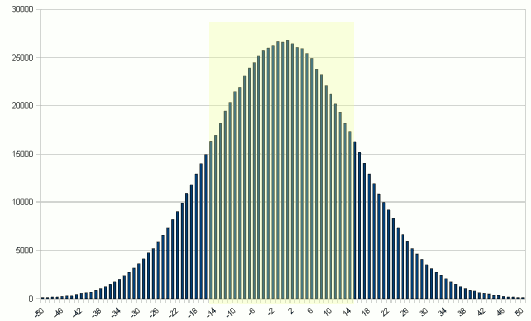
Random Nextgaussian

Weak Random Thesecurityvault
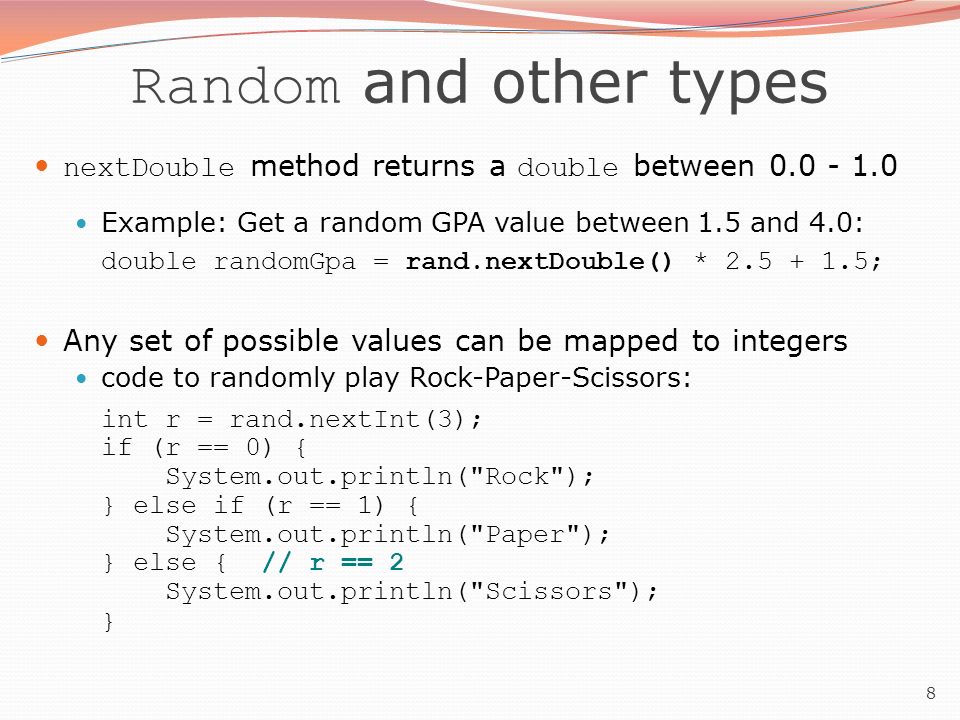
1 Building Java Programs Chapter 5 Lecture 5 2 Random Numbers Reading 5 1 Ppt Download
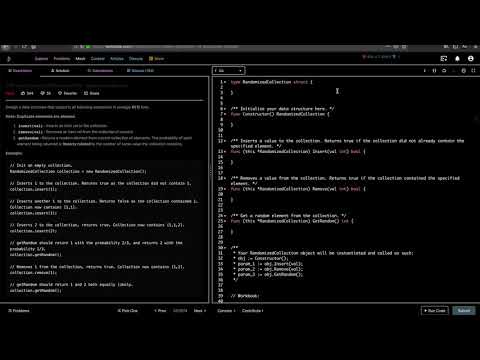
Clean O 1 Java Solution With Hashmap And Set Leetcode Discuss

Injecting Entropy In Your Code Make Sure That You Understand It By Rajneesh Aggarwal Dev Genius Jul Medium
2

Unable To Show Random Data From Firebase Realtime Database In Android Stack Overflow
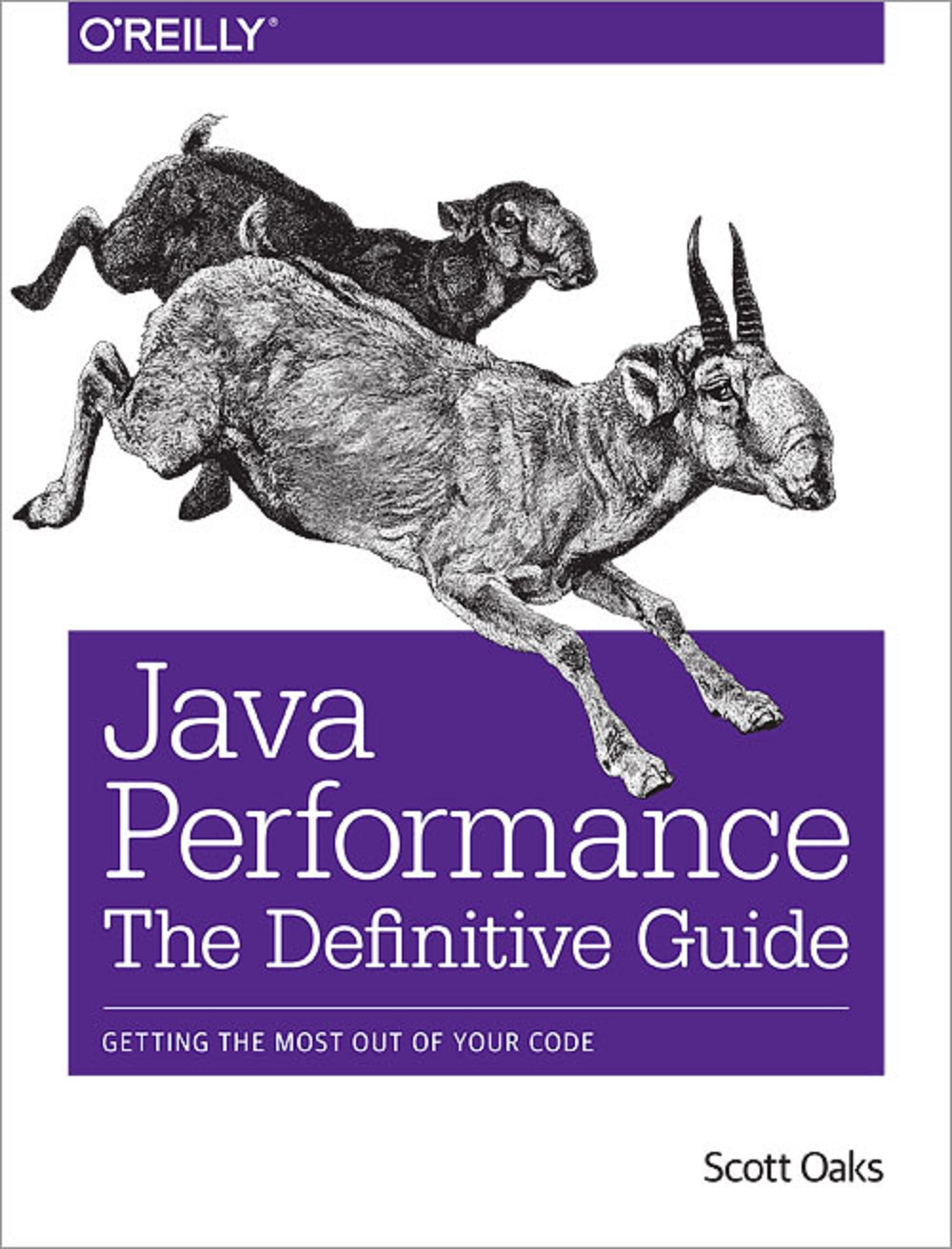
How To Generate Random Number Between 1 To 10 Java Example Java67
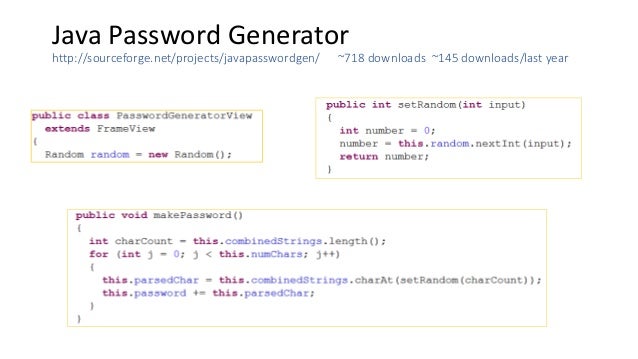
Cracking Pseudorandom Sequences Generators In Java Applications

Generating Randoms But A Few Minor Errors
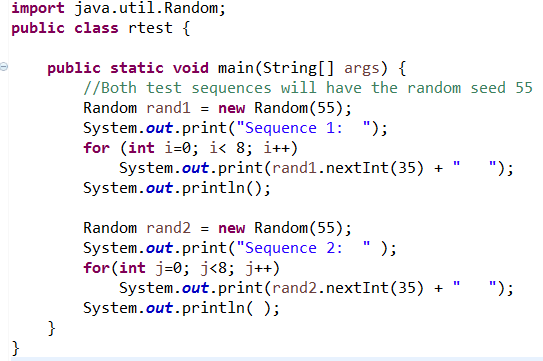
Java Random Generation Javabitsnotebook Com

Beginning Programming With Java For Dummies 4th Edition Pages 1 250 Text Version Fliphtml5
Q Tbn 3aand9gcsts6wtzf6uarueun8utygpnuqv6pxqsv2u Vkmupvngcimxwrd Usqp Cau
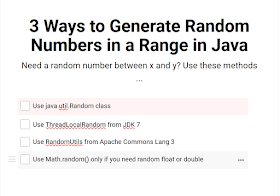
3 Ways To Create Random Numbers In A Range In Java Java67

Kotlin Mega Tutorial Superkotlin
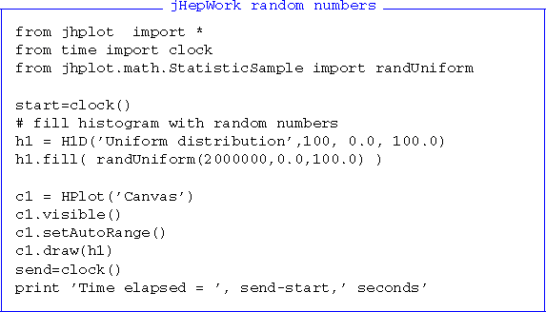
Random Numbers And Statistical Samples Springerlink
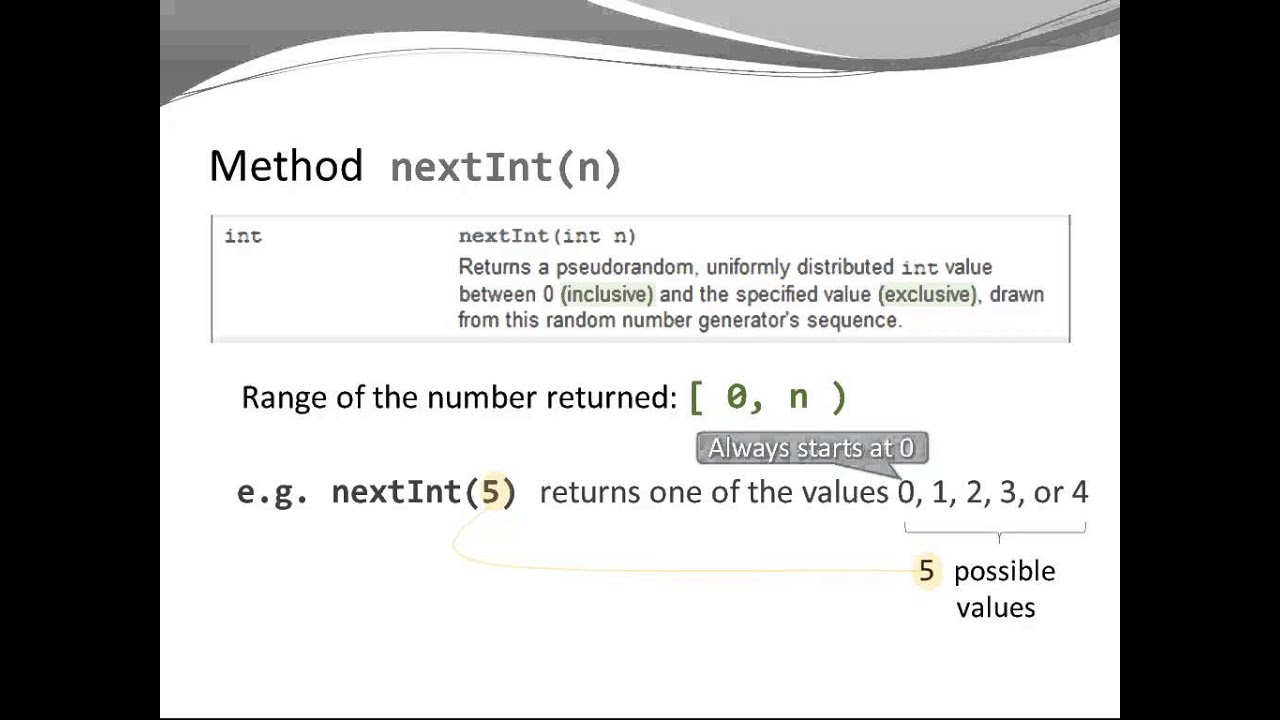
Java Basics Random Number Generation Part 1 Youtube
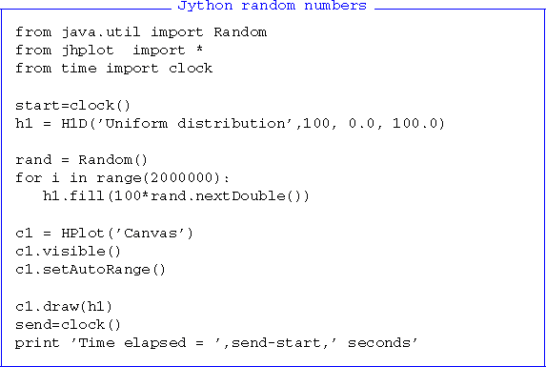
Random Numbers And Statistical Samples Springerlink
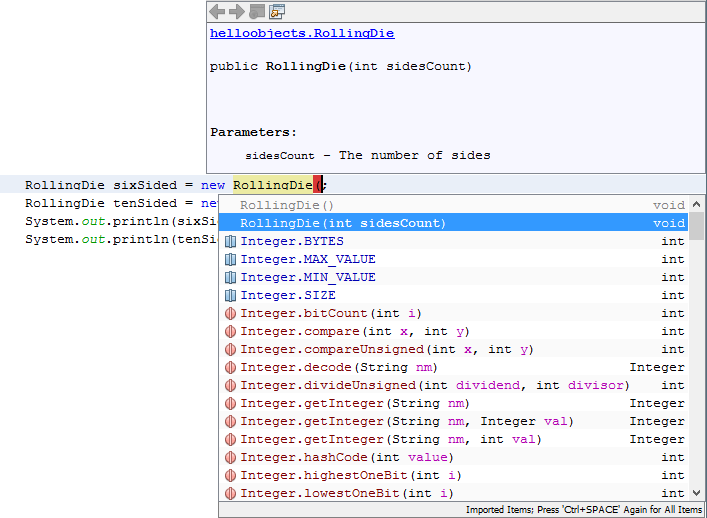
Lesson 3 Rollingdie In Java Constructors And Random Numbers
Http Horstmann Com Bigj Ap Bigj Guide Ch6 Pdf
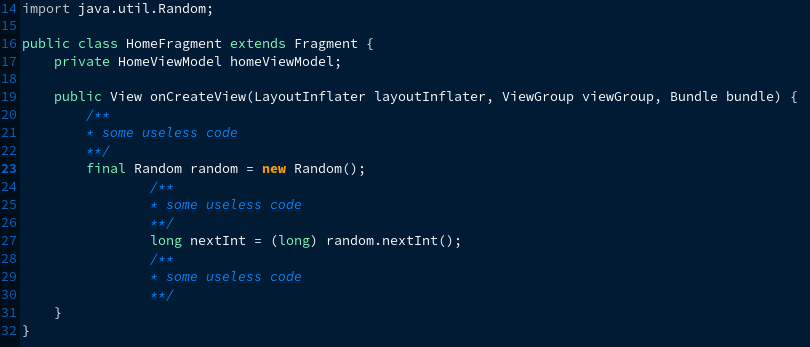
Casino Sharkyctf The Challenge Description Goes Like By yushman Choudhary Medium

Solved Write A Program That Creates A Random Object With Seed Chegg Com
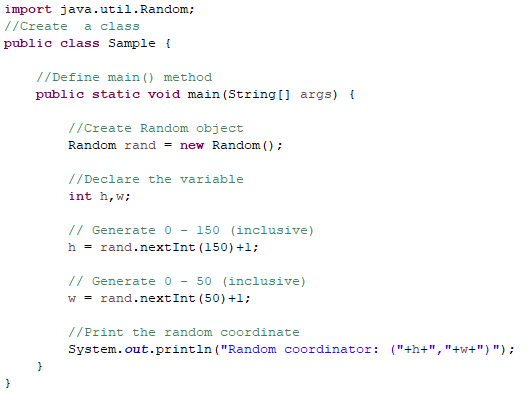
最高 Java Random Nextint
Courses Cs Washington Edu Courses Cse142 09su Lectures 09 07 22 Ch05 2 13 Ch05 2 Random Pdf

Random Number Program In Java Baldcirclenetworking

Intro To Java Midterm 2 Flashcards Quizlet

Java Random Tutorial Math Random Vs Random Class Nextint Nextdouble Youtube

Java Random Journaldev
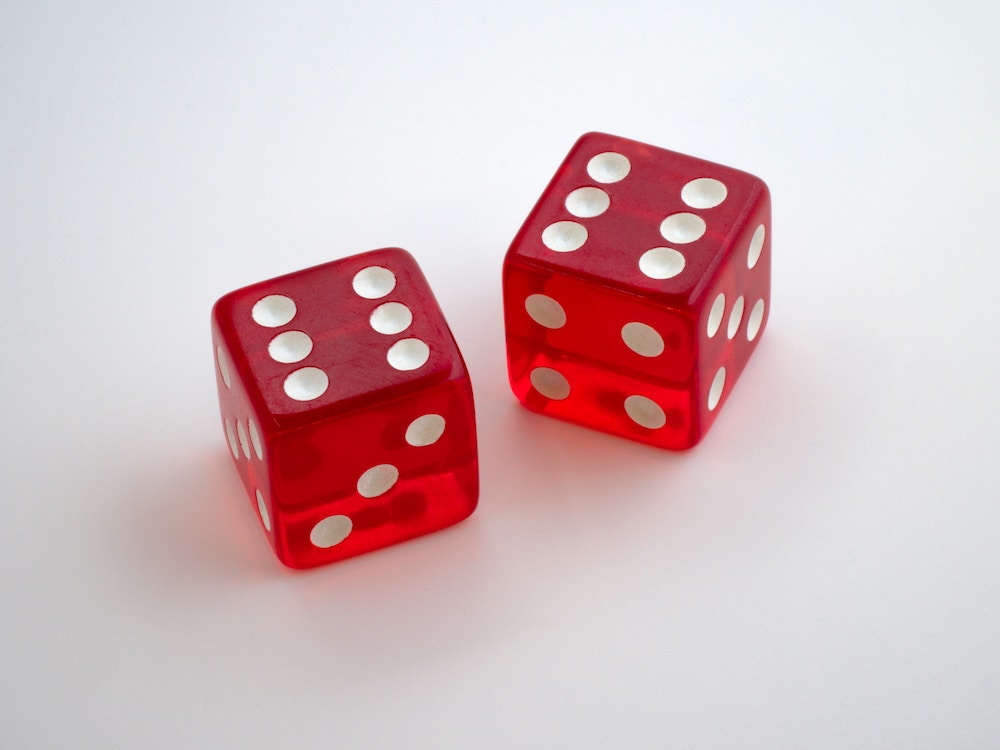
Random Number Generation With Java
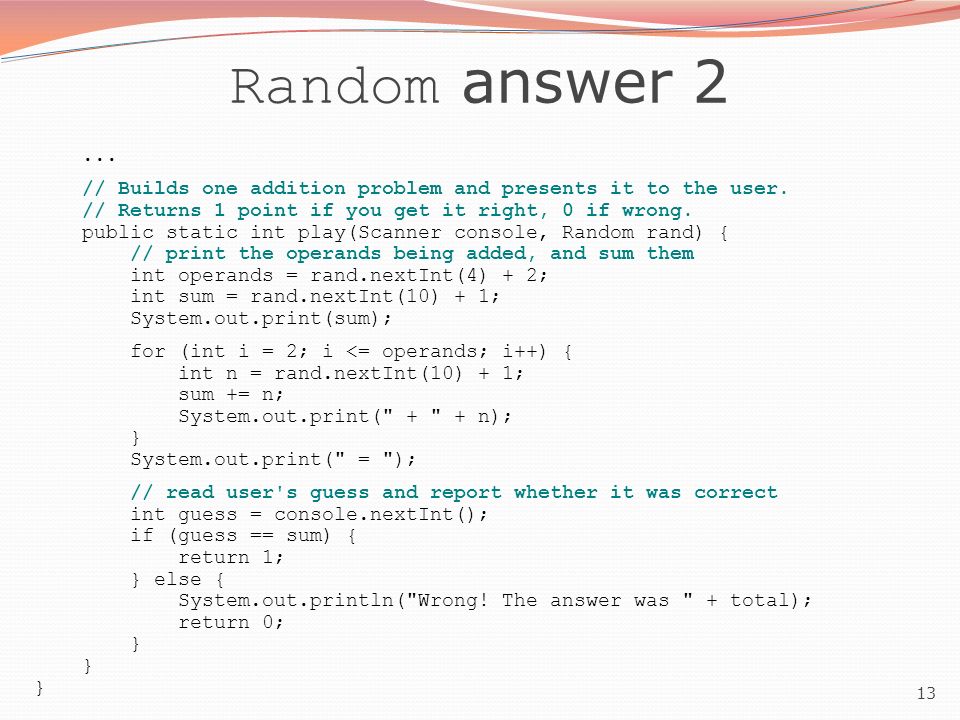
1 Building Java Programs Chapter 5 Lecture 5 2 Random Numbers Reading 5 1 Ppt Download

Java Random Is Always Delivering A Negative Trend On The Long Run Stack Overflow
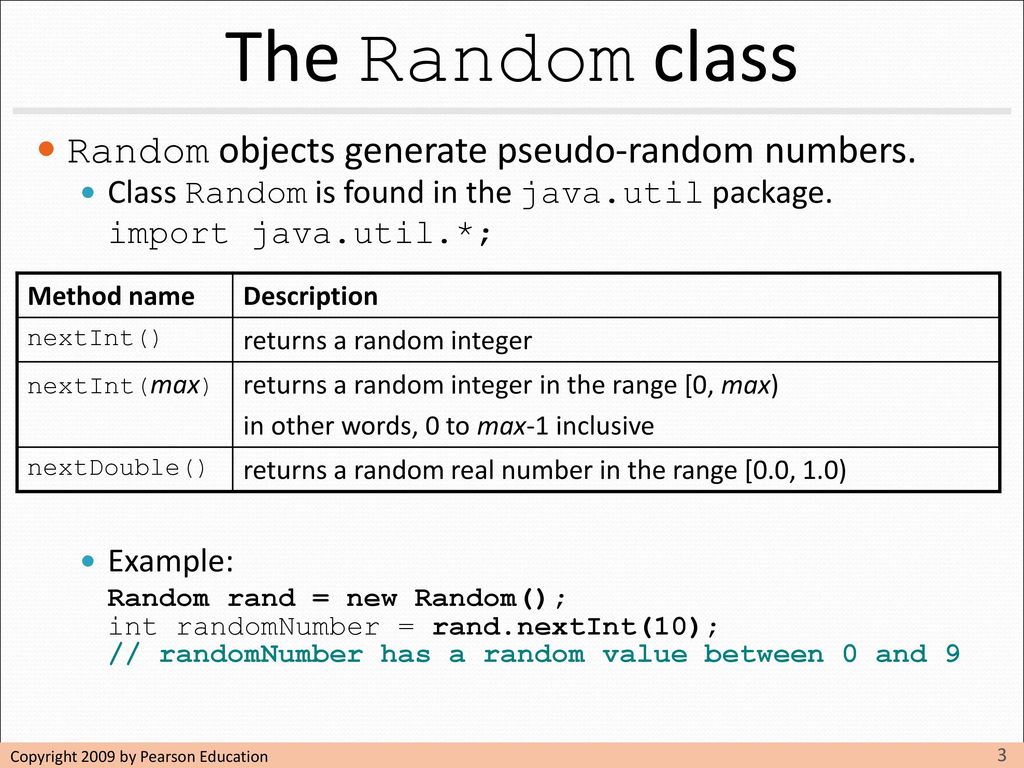
Building Java Programs Ppt Download
2

Beginning Java Programming For Dummies Flip Book Pages 351 400 Pubhtml5

A Puzzle From A Brief History Of The Java World And A Peek Forward Presented By Neal Gafter Java Brief Java Programming Language
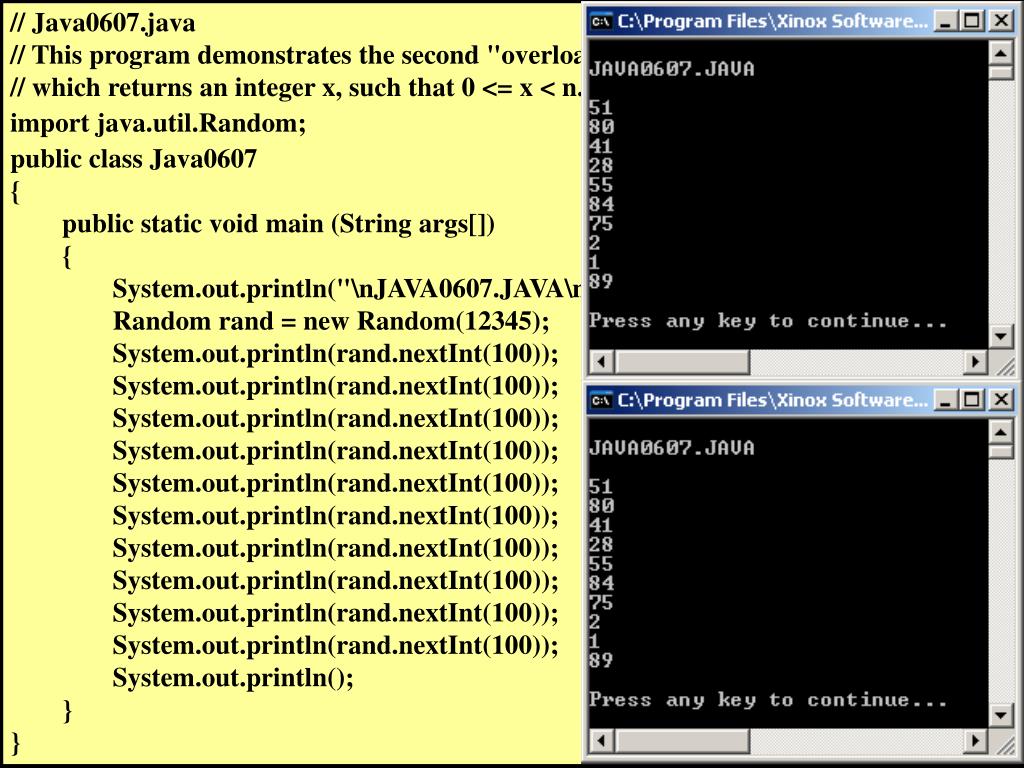
Ppt Chapter 6 Slides Powerpoint Presentation Free Download Id
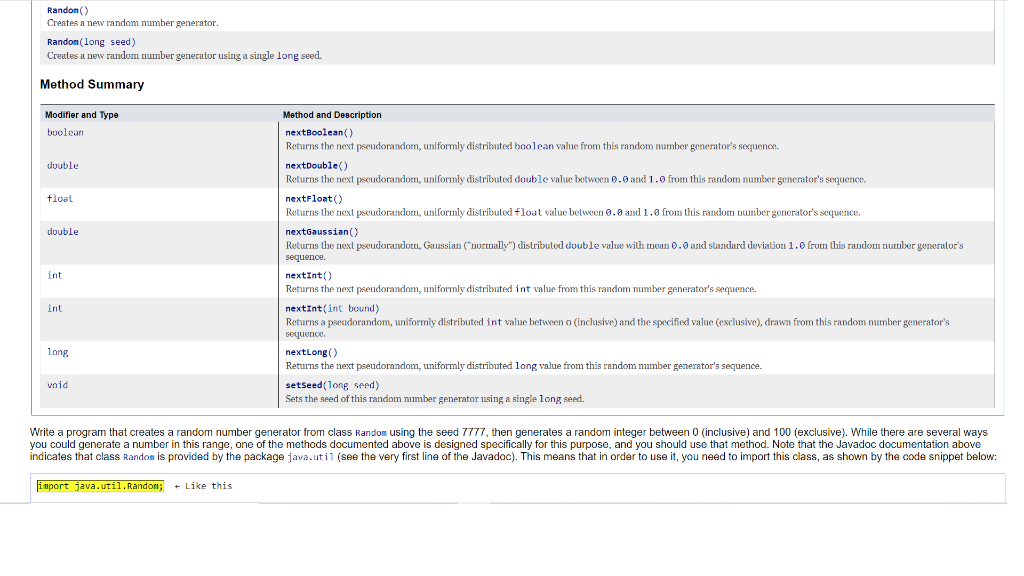
Solved Write A Program That Creates A Random Number Gener Chegg Com
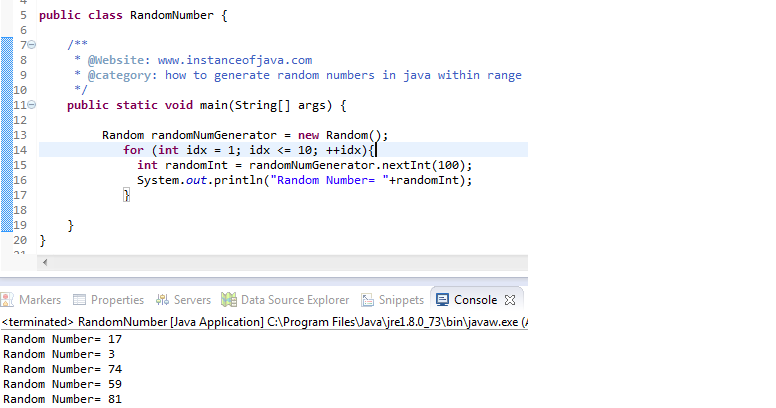
How To Generate Unique Random Numbers In Java Instanceofjava
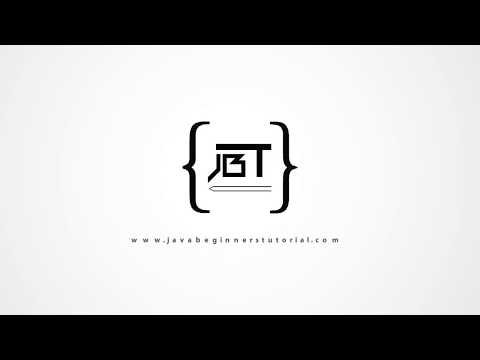
Generate Random Number In Java Java Beginners Tutorial
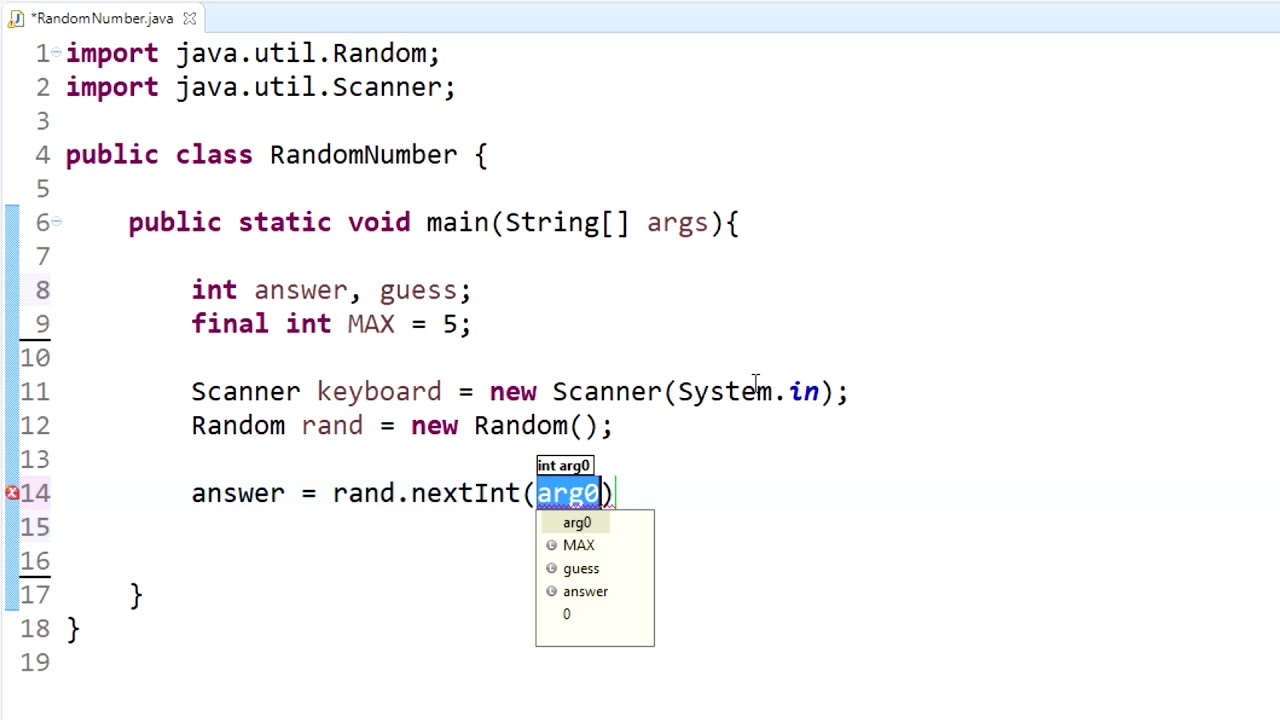
Java Programming Tutorial 10 Random Number Generator Number Guessing Game Youtube

Algorithm And Data Structure Java Implementation Binary Search Tree
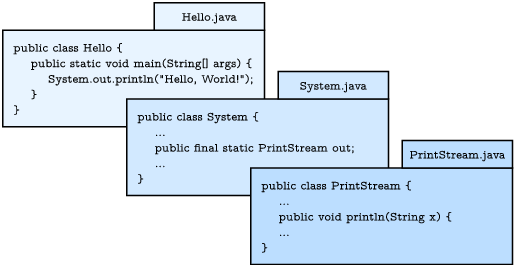
Input And Output Think Java Trinket

How To Find Random Numbers In A Range In Dart Codevscolor

Lab8 Doc Building Java Programs Chapter 8 Lab Handout Classes 1 Define A Class Named Randomwalker A Randomwalker Object Should Keep Track Of Its X Y Course Hero

Hazelcast Imdg For Developers Hazelcast
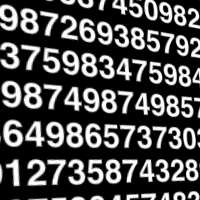
Java Return A Random Item From A List Mkyong Com
Solved Import Java Util Random Public Class Exercise5 Pu Chegg Com

Weak Random Thesecurityvault

Building Java Programs Chapter 5 Program Logic And Indefinite Loops Copyright C Pearson All Rights Reserved Ppt Download
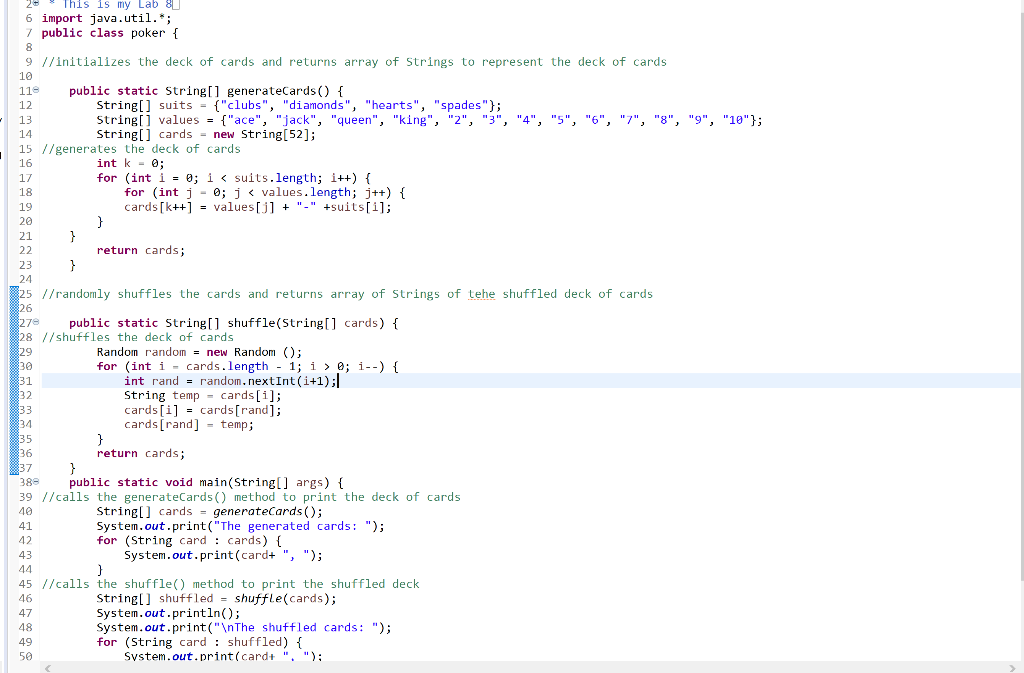
Solved Professor Says Line 31 Random Nextint I 1 Wil Chegg Com
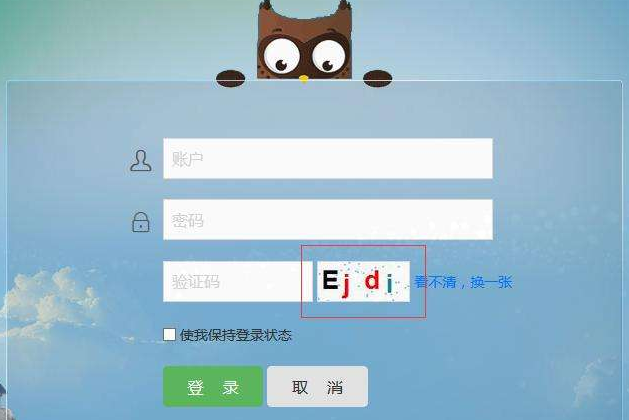
Program Life Three Language Implementation User Login Interface Random Verification Code Source Code Sharing
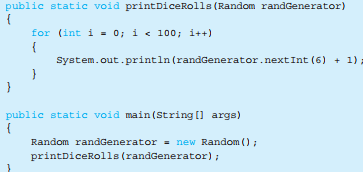
Solved The Following Is A Short Snippet Of Code That Simulates Rolling A 1 Answer Transtutors
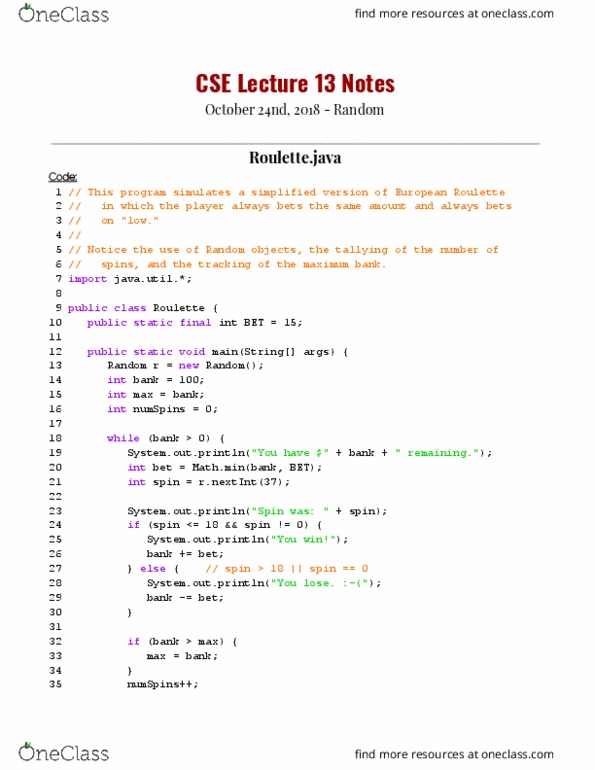
Cse 142 Lecture Reference Semantics Array Mystery Array Tallying Oneclass
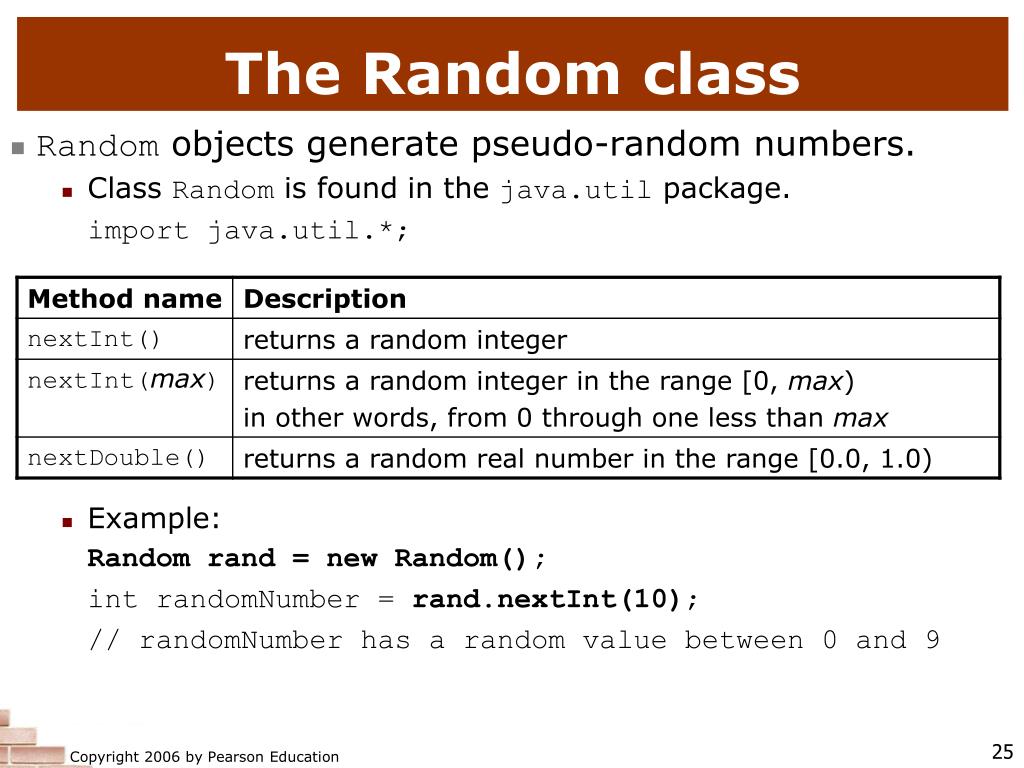
Ppt Building Java Programs Powerpoint Presentation Free Download Id
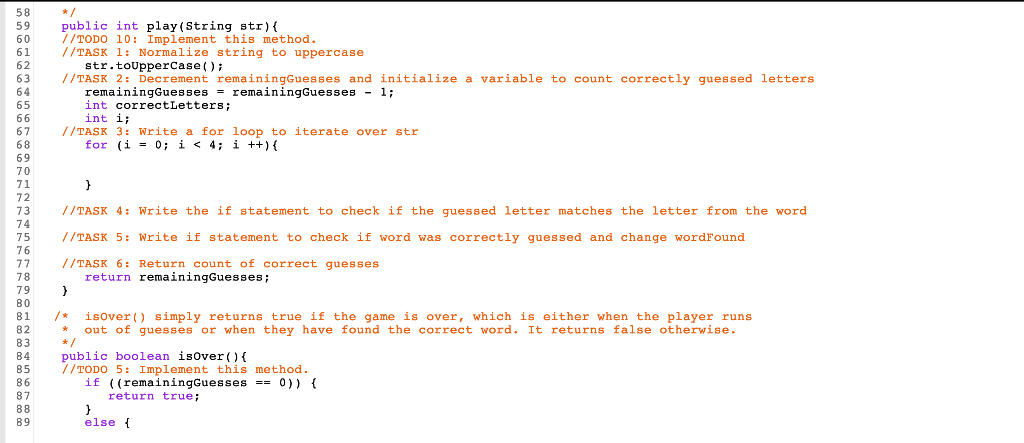
1 Remember To Import Java Util Random Othe Chegg Com
2

When I Use Intellij Idea To Develope Java Program There Is Always A Debug Configuration Window Then I Can T Even Coding Stack Overflow
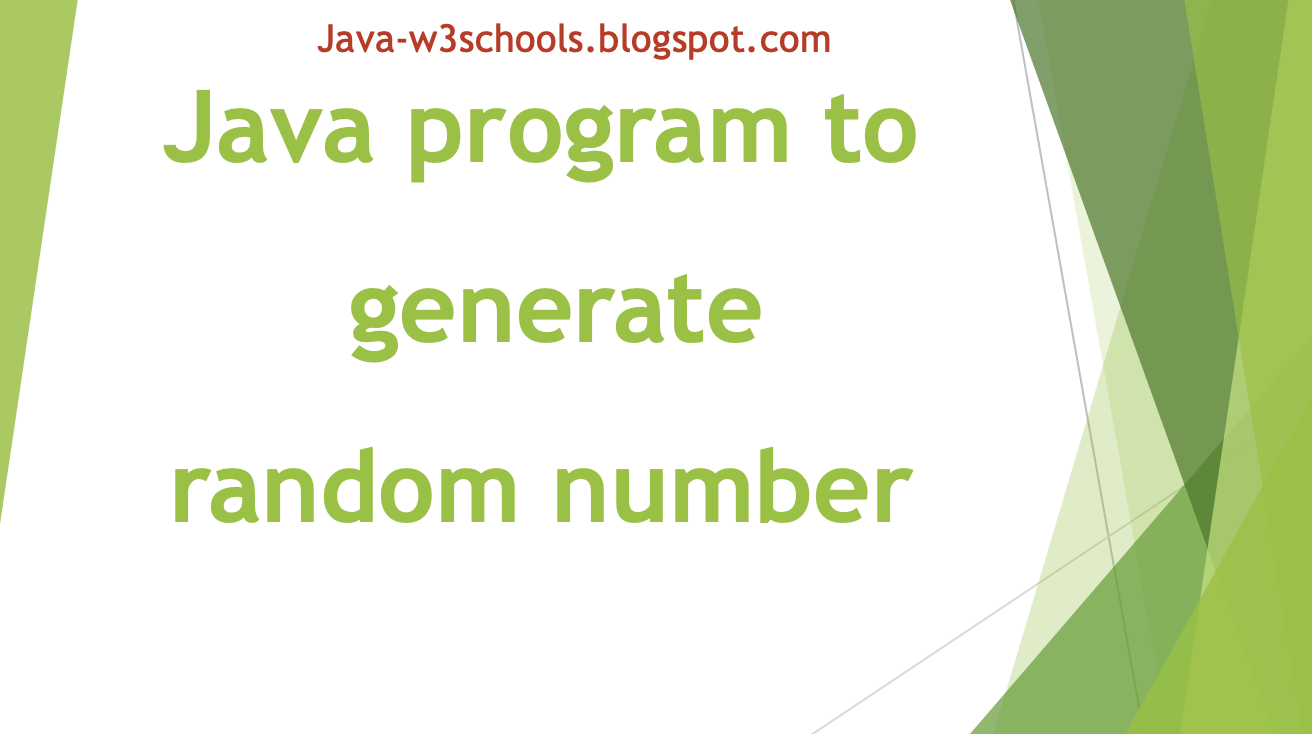
Java Program To Generate Random Number Using Random Nextint Math Random And Threadlocalrandom Javaprogramto Com

Building Java Programs Chapter 5 Ppt Download
1
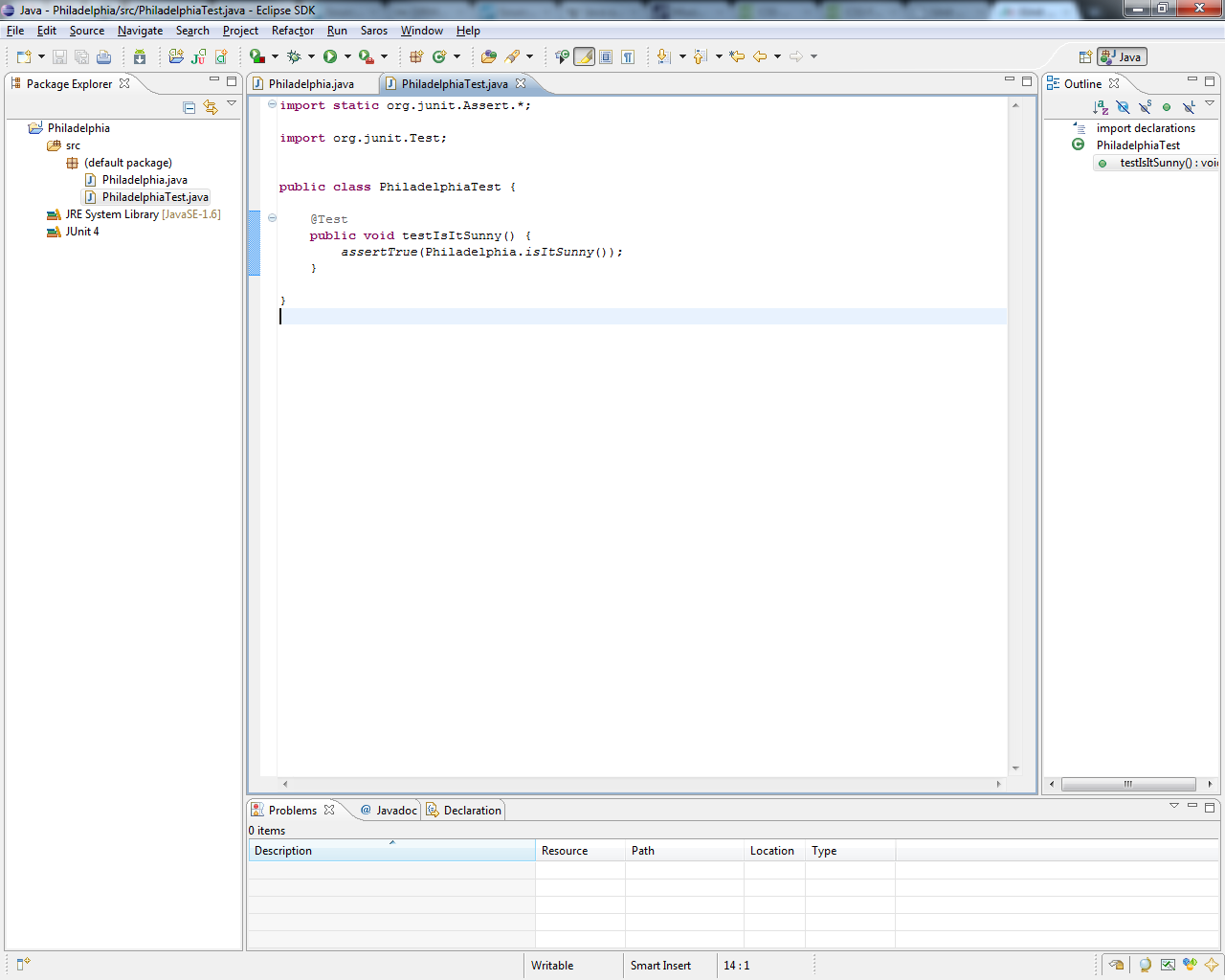
Unit Testing

最高 Java Random Nextint

How To Generate Random Integers In Java Learning Journal
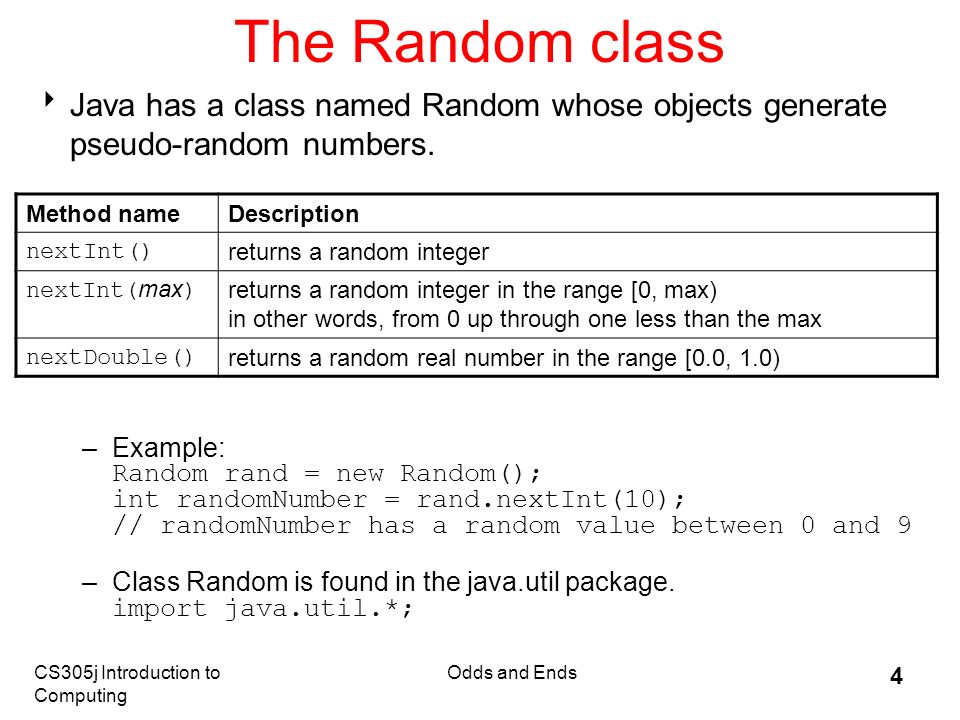
Cs305j Introduction To Computing Odds And Ends 1 Topic 16 Creating Correct Programs It Is A Profoundly Erroneous Truism Repeated By All The Copybooks Ppt Download

Java Object Random Always Returns Error Random Nextint Int Line Not Available Stack Overflow
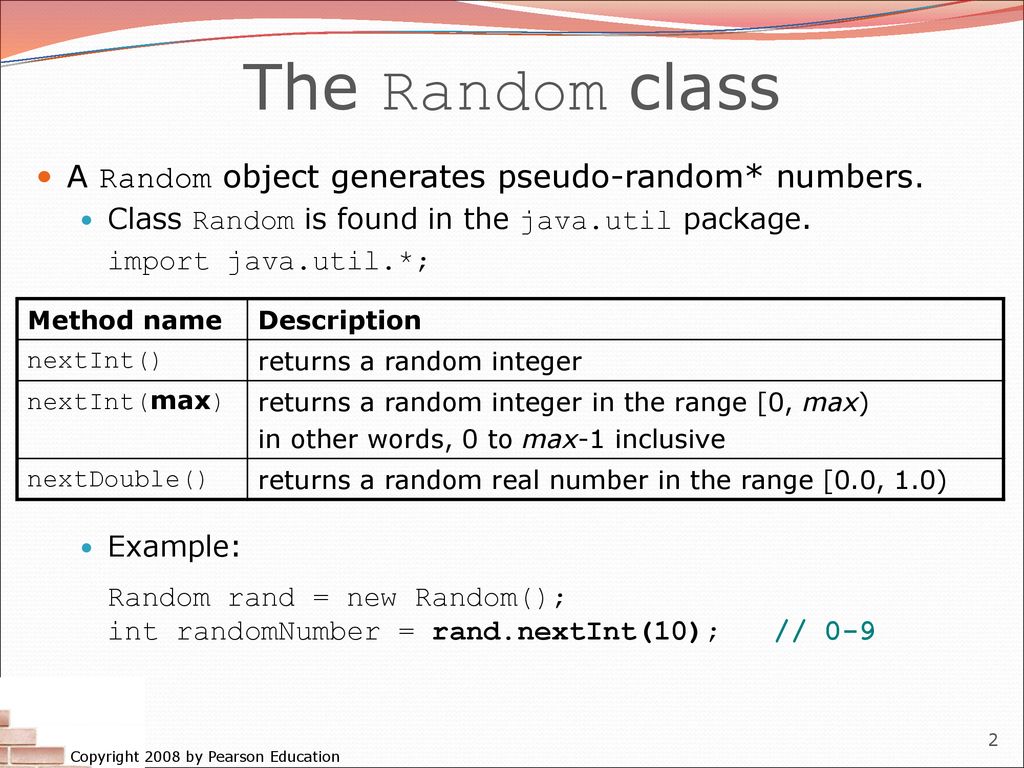
Building Java Programs Ppt Download
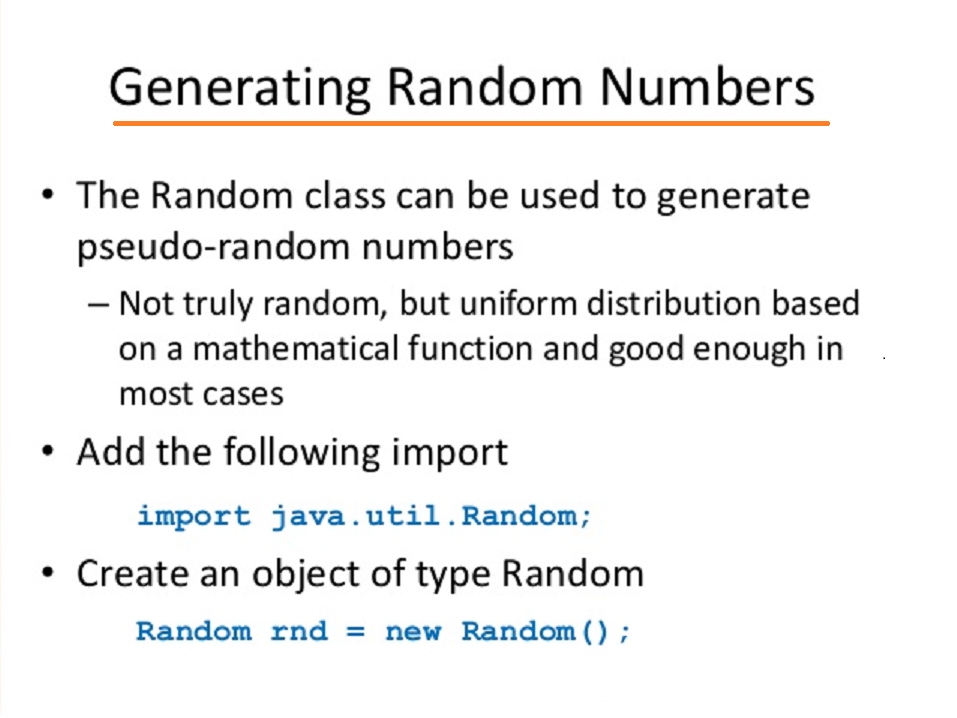
How To Generate Random Number Between 1 To 10 Java Example Java67
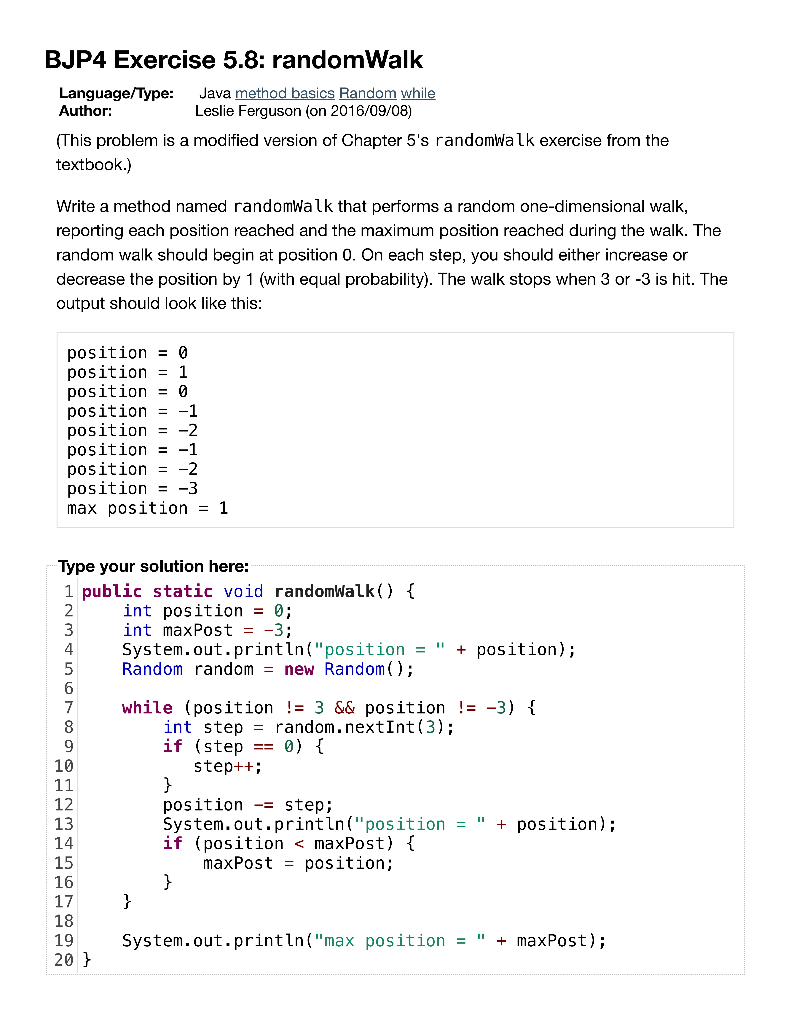
Solved Bjp4 Exercise 5 8 Random Walk Language Type Java Chegg Com

How To Find Random Numbers In A Range In Dart Codevscolor